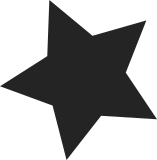
This passes the errata fix using a GPIO to control the RTS pin on one of the AT91 chips to use gpiolib instead of the AT91-specific interfaces. Also remove the reliance on compile-time #defines and the cpu_* check and rely on the platform passing down the proper GPIO pin through platform data. This is a prerequisite for getting rid of the local GPIO implementation in the AT91 platform and move toward multiplatform. The patch also adds device tree support for getting the RTS GPIO pin from the device tree on DT boot paths. Signed-off-by: Nicolas Ferre <nicolas.ferre@atmel.com> Acked-by: Jean-Christophe PLAGNIOL-VILLARD <plagnioj@jcrosoft.com> Signed-off-by: Linus Walleij <linus.walleij@linaro.org> Acked-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Signed-off-by: Uwe Kleine-König <u.kleine-koenig@pengutronix.de>
106 lines
2.8 KiB
C
106 lines
2.8 KiB
C
/*
|
|
* atmel platform data
|
|
*
|
|
* GPL v2 Only
|
|
*/
|
|
|
|
#ifndef __ATMEL_H__
|
|
#define __ATMEL_H__
|
|
|
|
#include <linux/mtd/nand.h>
|
|
#include <linux/mtd/partitions.h>
|
|
#include <linux/device.h>
|
|
#include <linux/i2c.h>
|
|
#include <linux/leds.h>
|
|
#include <linux/spi/spi.h>
|
|
#include <linux/usb/atmel_usba_udc.h>
|
|
#include <linux/atmel-mci.h>
|
|
#include <sound/atmel-ac97c.h>
|
|
#include <linux/serial.h>
|
|
#include <linux/platform_data/macb.h>
|
|
|
|
/*
|
|
* at91: 6 USARTs and one DBGU port (SAM9260)
|
|
* avr32: 4
|
|
*/
|
|
#define ATMEL_MAX_UART 7
|
|
|
|
/* USB Device */
|
|
struct at91_udc_data {
|
|
int vbus_pin; /* high == host powering us */
|
|
u8 vbus_active_low; /* vbus polarity */
|
|
u8 vbus_polled; /* Use polling, not interrupt */
|
|
int pullup_pin; /* active == D+ pulled up */
|
|
u8 pullup_active_low; /* true == pullup_pin is active low */
|
|
};
|
|
|
|
/* Compact Flash */
|
|
struct at91_cf_data {
|
|
int irq_pin; /* I/O IRQ */
|
|
int det_pin; /* Card detect */
|
|
int vcc_pin; /* power switching */
|
|
int rst_pin; /* card reset */
|
|
u8 chipselect; /* EBI Chip Select number */
|
|
u8 flags;
|
|
#define AT91_CF_TRUE_IDE 0x01
|
|
#define AT91_IDE_SWAP_A0_A2 0x02
|
|
};
|
|
|
|
/* USB Host */
|
|
#define AT91_MAX_USBH_PORTS 3
|
|
struct at91_usbh_data {
|
|
int vbus_pin[AT91_MAX_USBH_PORTS]; /* port power-control pin */
|
|
int overcurrent_pin[AT91_MAX_USBH_PORTS];
|
|
u8 ports; /* number of ports on root hub */
|
|
u8 overcurrent_supported;
|
|
u8 vbus_pin_active_low[AT91_MAX_USBH_PORTS];
|
|
u8 overcurrent_status[AT91_MAX_USBH_PORTS];
|
|
u8 overcurrent_changed[AT91_MAX_USBH_PORTS];
|
|
};
|
|
|
|
/* NAND / SmartMedia */
|
|
struct atmel_nand_data {
|
|
int enable_pin; /* chip enable */
|
|
int det_pin; /* card detect */
|
|
int rdy_pin; /* ready/busy */
|
|
u8 rdy_pin_active_low; /* rdy_pin value is inverted */
|
|
u8 ale; /* address line number connected to ALE */
|
|
u8 cle; /* address line number connected to CLE */
|
|
u8 bus_width_16; /* buswidth is 16 bit */
|
|
u8 ecc_mode; /* ecc mode */
|
|
u8 on_flash_bbt; /* bbt on flash */
|
|
struct mtd_partition *parts;
|
|
unsigned int num_parts;
|
|
bool has_dma; /* support dma transfer */
|
|
|
|
/* default is false, only for at32ap7000 chip is true */
|
|
bool need_reset_workaround;
|
|
};
|
|
|
|
/* Serial */
|
|
struct atmel_uart_data {
|
|
int num; /* port num */
|
|
short use_dma_tx; /* use transmit DMA? */
|
|
short use_dma_rx; /* use receive DMA? */
|
|
void __iomem *regs; /* virt. base address, if any */
|
|
struct serial_rs485 rs485; /* rs485 settings */
|
|
int rts_gpio; /* optional RTS GPIO */
|
|
};
|
|
|
|
/* Touchscreen Controller */
|
|
struct at91_tsadcc_data {
|
|
unsigned int adc_clock;
|
|
u8 pendet_debounce;
|
|
u8 ts_sample_hold_time;
|
|
};
|
|
|
|
/* CAN */
|
|
struct at91_can_data {
|
|
void (*transceiver_switch)(int on);
|
|
};
|
|
|
|
/* FIXME: this needs a better location, but gets stuff building again */
|
|
extern int at91_suspend_entering_slow_clock(void);
|
|
|
|
#endif /* __ATMEL_H__ */
|