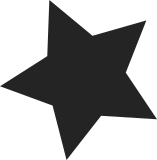
There are good reasons to supports helpers in user-space instead: * Rapid connection tracking helper development, as developing code in user-space is usually faster. * Reliability: A buggy helper does not crash the kernel. Moreover, we can monitor the helper process and restart it in case of problems. * Security: Avoid complex string matching and mangling in kernel-space running in privileged mode. Going further, we can even think about running user-space helpers as a non-root process. * Extensibility: It allows the development of very specific helpers (most likely non-standard proprietary protocols) that are very likely not to be accepted for mainline inclusion in the form of kernel-space connection tracking helpers. This patch adds the infrastructure to allow the implementation of user-space conntrack helpers by means of the new nfnetlink subsystem `nfnetlink_cthelper' and the existing queueing infrastructure (nfnetlink_queue). I had to add the new hook NF_IP6_PRI_CONNTRACK_HELPER to register ipv[4|6]_helper which results from splitting ipv[4|6]_confirm into two pieces. This change is required not to break NAT sequence adjustment and conntrack confirmation for traffic that is enqueued to our user-space conntrack helpers. Basic operation, in a few steps: 1) Register user-space helper by means of `nfct': nfct helper add ftp inet tcp [ It must be a valid existing helper supported by conntrack-tools ] 2) Add rules to enable the FTP user-space helper which is used to track traffic going to TCP port 21. For locally generated packets: iptables -I OUTPUT -t raw -p tcp --dport 21 -j CT --helper ftp For non-locally generated packets: iptables -I PREROUTING -t raw -p tcp --dport 21 -j CT --helper ftp 3) Run the test conntrackd in helper mode (see example files under doc/helper/conntrackd.conf conntrackd 4) Generate FTP traffic going, if everything is OK, then conntrackd should create expectations (you can check that with `conntrack': conntrack -E expect [NEW] 301 proto=6 src=192.168.1.136 dst=130.89.148.12 sport=0 dport=54037 mask-src=255.255.255.255 mask-dst=255.255.255.255 sport=0 dport=65535 master-src=192.168.1.136 master-dst=130.89.148.12 sport=57127 dport=21 class=0 helper=ftp [DESTROY] 301 proto=6 src=192.168.1.136 dst=130.89.148.12 sport=0 dport=54037 mask-src=255.255.255.255 mask-dst=255.255.255.255 sport=0 dport=65535 master-src=192.168.1.136 master-dst=130.89.148.12 sport=57127 dport=21 class=0 helper=ftp This confirms that our test helper is receiving packets including the conntrack information, and adding expectations in kernel-space. The user-space helper can also store its private tracking information in the conntrack structure in the kernel via the CTA_HELP_INFO. The kernel will consider this a binary blob whose layout is unknown. This information will be included in the information that is transfered to user-space via glue code that integrates nfnetlink_queue and ctnetlink. Signed-off-by: Pablo Neira Ayuso <pablo@netfilter.org>
95 lines
2.6 KiB
C
95 lines
2.6 KiB
C
#ifndef __LINUX_IP6_NETFILTER_H
|
|
#define __LINUX_IP6_NETFILTER_H
|
|
|
|
/* IPv6-specific defines for netfilter.
|
|
* (C)1998 Rusty Russell -- This code is GPL.
|
|
* (C)1999 David Jeffery
|
|
* this header was blatantly ripped from netfilter_ipv4.h
|
|
* it's amazing what adding a bunch of 6s can do =8^)
|
|
*/
|
|
|
|
#include <linux/netfilter.h>
|
|
|
|
/* only for userspace compatibility */
|
|
#ifndef __KERNEL__
|
|
|
|
#include <limits.h> /* for INT_MIN, INT_MAX */
|
|
|
|
/* IP Cache bits. */
|
|
/* Src IP address. */
|
|
#define NFC_IP6_SRC 0x0001
|
|
/* Dest IP address. */
|
|
#define NFC_IP6_DST 0x0002
|
|
/* Input device. */
|
|
#define NFC_IP6_IF_IN 0x0004
|
|
/* Output device. */
|
|
#define NFC_IP6_IF_OUT 0x0008
|
|
/* TOS. */
|
|
#define NFC_IP6_TOS 0x0010
|
|
/* Protocol. */
|
|
#define NFC_IP6_PROTO 0x0020
|
|
/* IP options. */
|
|
#define NFC_IP6_OPTIONS 0x0040
|
|
/* Frag & flags. */
|
|
#define NFC_IP6_FRAG 0x0080
|
|
|
|
|
|
/* Per-protocol information: only matters if proto match. */
|
|
/* TCP flags. */
|
|
#define NFC_IP6_TCPFLAGS 0x0100
|
|
/* Source port. */
|
|
#define NFC_IP6_SRC_PT 0x0200
|
|
/* Dest port. */
|
|
#define NFC_IP6_DST_PT 0x0400
|
|
/* Something else about the proto */
|
|
#define NFC_IP6_PROTO_UNKNOWN 0x2000
|
|
|
|
/* IP6 Hooks */
|
|
/* After promisc drops, checksum checks. */
|
|
#define NF_IP6_PRE_ROUTING 0
|
|
/* If the packet is destined for this box. */
|
|
#define NF_IP6_LOCAL_IN 1
|
|
/* If the packet is destined for another interface. */
|
|
#define NF_IP6_FORWARD 2
|
|
/* Packets coming from a local process. */
|
|
#define NF_IP6_LOCAL_OUT 3
|
|
/* Packets about to hit the wire. */
|
|
#define NF_IP6_POST_ROUTING 4
|
|
#define NF_IP6_NUMHOOKS 5
|
|
#endif /* ! __KERNEL__ */
|
|
|
|
|
|
enum nf_ip6_hook_priorities {
|
|
NF_IP6_PRI_FIRST = INT_MIN,
|
|
NF_IP6_PRI_CONNTRACK_DEFRAG = -400,
|
|
NF_IP6_PRI_RAW = -300,
|
|
NF_IP6_PRI_SELINUX_FIRST = -225,
|
|
NF_IP6_PRI_CONNTRACK = -200,
|
|
NF_IP6_PRI_MANGLE = -150,
|
|
NF_IP6_PRI_NAT_DST = -100,
|
|
NF_IP6_PRI_FILTER = 0,
|
|
NF_IP6_PRI_SECURITY = 50,
|
|
NF_IP6_PRI_NAT_SRC = 100,
|
|
NF_IP6_PRI_SELINUX_LAST = 225,
|
|
NF_IP6_PRI_CONNTRACK_HELPER = 300,
|
|
NF_IP6_PRI_LAST = INT_MAX,
|
|
};
|
|
|
|
#ifdef __KERNEL__
|
|
|
|
#ifdef CONFIG_NETFILTER
|
|
extern int ip6_route_me_harder(struct sk_buff *skb);
|
|
extern __sum16 nf_ip6_checksum(struct sk_buff *skb, unsigned int hook,
|
|
unsigned int dataoff, u_int8_t protocol);
|
|
|
|
extern int ipv6_netfilter_init(void);
|
|
extern void ipv6_netfilter_fini(void);
|
|
#else /* CONFIG_NETFILTER */
|
|
static inline int ipv6_netfilter_init(void) { return 0; }
|
|
static inline void ipv6_netfilter_fini(void) { return; }
|
|
#endif /* CONFIG_NETFILTER */
|
|
|
|
#endif /* __KERNEL__ */
|
|
|
|
#endif /*__LINUX_IP6_NETFILTER_H*/
|