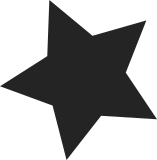
Fix potential use of uninitialised variable caused by recent decompressor code optimisations. In zlib_uncompress (zlib_wrapper.c) we have int zlib_err, zlib_init = 0; ... do { ... if (avail == 0) { offset = 0; put_bh(bh[k++]); continue; } ... zlib_err = zlib_inflate(stream, Z_SYNC_FLUSH); ... } while (zlib_err == Z_OK); If continue is executed (avail == 0) then the while condition will be evaluated testing zlib_err, which is uninitialised first time around the loop. Fix this by getting rid of the 'if (avail == 0)' condition test, this edge condition should not be being handled in the decompressor code, and instead handle it generically in the caller code. Similarly for xz_wrapper.c. Incidentally, on most architectures (bar Mips and Parisc), no uninitialised variable warning is generated by gcc, this is because the while condition test on continue is optimised out and not performed (when executing continue zlib_err has not been changed since entering the loop, and logically if the while condition was true previously, then it's still true). Signed-off-by: Phillip Lougher <phillip@lougher.demon.co.uk> Reported-by: Jesper Juhl <jj@chaosbits.net> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
148 lines
3.5 KiB
C
148 lines
3.5 KiB
C
/*
|
|
* Squashfs - a compressed read only filesystem for Linux
|
|
*
|
|
* Copyright (c) 2002, 2003, 2004, 2005, 2006, 2007, 2008, 2009, 2010
|
|
* Phillip Lougher <phillip@lougher.demon.co.uk>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version 2,
|
|
* or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
*
|
|
* xz_wrapper.c
|
|
*/
|
|
|
|
|
|
#include <linux/mutex.h>
|
|
#include <linux/buffer_head.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/xz.h>
|
|
|
|
#include "squashfs_fs.h"
|
|
#include "squashfs_fs_sb.h"
|
|
#include "squashfs_fs_i.h"
|
|
#include "squashfs.h"
|
|
#include "decompressor.h"
|
|
|
|
struct squashfs_xz {
|
|
struct xz_dec *state;
|
|
struct xz_buf buf;
|
|
};
|
|
|
|
static void *squashfs_xz_init(struct squashfs_sb_info *msblk)
|
|
{
|
|
int block_size = max_t(int, msblk->block_size, SQUASHFS_METADATA_SIZE);
|
|
|
|
struct squashfs_xz *stream = kmalloc(sizeof(*stream), GFP_KERNEL);
|
|
if (stream == NULL)
|
|
goto failed;
|
|
|
|
stream->state = xz_dec_init(XZ_PREALLOC, block_size);
|
|
if (stream->state == NULL)
|
|
goto failed;
|
|
|
|
return stream;
|
|
|
|
failed:
|
|
ERROR("Failed to allocate xz workspace\n");
|
|
kfree(stream);
|
|
return NULL;
|
|
}
|
|
|
|
|
|
static void squashfs_xz_free(void *strm)
|
|
{
|
|
struct squashfs_xz *stream = strm;
|
|
|
|
if (stream) {
|
|
xz_dec_end(stream->state);
|
|
kfree(stream);
|
|
}
|
|
}
|
|
|
|
|
|
static int squashfs_xz_uncompress(struct squashfs_sb_info *msblk, void **buffer,
|
|
struct buffer_head **bh, int b, int offset, int length, int srclength,
|
|
int pages)
|
|
{
|
|
enum xz_ret xz_err;
|
|
int avail, total = 0, k = 0, page = 0;
|
|
struct squashfs_xz *stream = msblk->stream;
|
|
|
|
mutex_lock(&msblk->read_data_mutex);
|
|
|
|
xz_dec_reset(stream->state);
|
|
stream->buf.in_pos = 0;
|
|
stream->buf.in_size = 0;
|
|
stream->buf.out_pos = 0;
|
|
stream->buf.out_size = PAGE_CACHE_SIZE;
|
|
stream->buf.out = buffer[page++];
|
|
|
|
do {
|
|
if (stream->buf.in_pos == stream->buf.in_size && k < b) {
|
|
avail = min(length, msblk->devblksize - offset);
|
|
length -= avail;
|
|
wait_on_buffer(bh[k]);
|
|
if (!buffer_uptodate(bh[k]))
|
|
goto release_mutex;
|
|
|
|
stream->buf.in = bh[k]->b_data + offset;
|
|
stream->buf.in_size = avail;
|
|
stream->buf.in_pos = 0;
|
|
offset = 0;
|
|
}
|
|
|
|
if (stream->buf.out_pos == stream->buf.out_size
|
|
&& page < pages) {
|
|
stream->buf.out = buffer[page++];
|
|
stream->buf.out_pos = 0;
|
|
total += PAGE_CACHE_SIZE;
|
|
}
|
|
|
|
xz_err = xz_dec_run(stream->state, &stream->buf);
|
|
|
|
if (stream->buf.in_pos == stream->buf.in_size && k < b)
|
|
put_bh(bh[k++]);
|
|
} while (xz_err == XZ_OK);
|
|
|
|
if (xz_err != XZ_STREAM_END) {
|
|
ERROR("xz_dec_run error, data probably corrupt\n");
|
|
goto release_mutex;
|
|
}
|
|
|
|
if (k < b) {
|
|
ERROR("xz_uncompress error, input remaining\n");
|
|
goto release_mutex;
|
|
}
|
|
|
|
total += stream->buf.out_pos;
|
|
mutex_unlock(&msblk->read_data_mutex);
|
|
return total;
|
|
|
|
release_mutex:
|
|
mutex_unlock(&msblk->read_data_mutex);
|
|
|
|
for (; k < b; k++)
|
|
put_bh(bh[k]);
|
|
|
|
return -EIO;
|
|
}
|
|
|
|
const struct squashfs_decompressor squashfs_xz_comp_ops = {
|
|
.init = squashfs_xz_init,
|
|
.free = squashfs_xz_free,
|
|
.decompress = squashfs_xz_uncompress,
|
|
.id = XZ_COMPRESSION,
|
|
.name = "xz",
|
|
.supported = 1
|
|
};
|