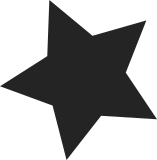
Use LLC for the receive path of Spanning Tree Protocol packets. This allows link local multicast packets to be received by other protocols (if they care), and uses the existing LLC code to get STP packets back into bridge code. The bridge multicast address is also checked, so bridges using other link local multicast addresses are ignored. This allows for use of different multicast addresses to define separate STP domains. Signed-off-by: Stephen Hemminger <shemminger@osdl.org> Signed-off-by: David S. Miller <davem@davemloft.net>
83 lines
1.7 KiB
C
83 lines
1.7 KiB
C
/*
|
|
* Generic parts
|
|
* Linux ethernet bridge
|
|
*
|
|
* Authors:
|
|
* Lennert Buytenhek <buytenh@gnu.org>
|
|
*
|
|
* $Id: br.c,v 1.47 2001/12/24 00:56:41 davem Exp $
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/config.h>
|
|
#include <linux/module.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/netdevice.h>
|
|
#include <linux/etherdevice.h>
|
|
#include <linux/init.h>
|
|
#include <linux/llc.h>
|
|
#include <net/llc.h>
|
|
|
|
#include "br_private.h"
|
|
|
|
int (*br_should_route_hook) (struct sk_buff **pskb) = NULL;
|
|
|
|
static struct llc_sap *br_stp_sap;
|
|
|
|
static int __init br_init(void)
|
|
{
|
|
br_stp_sap = llc_sap_open(LLC_SAP_BSPAN, br_stp_rcv);
|
|
if (!br_stp_sap) {
|
|
printk(KERN_ERR "bridge: can't register sap for STP\n");
|
|
return -EBUSY;
|
|
}
|
|
|
|
br_fdb_init();
|
|
|
|
#ifdef CONFIG_BRIDGE_NETFILTER
|
|
if (br_netfilter_init())
|
|
return 1;
|
|
#endif
|
|
brioctl_set(br_ioctl_deviceless_stub);
|
|
br_handle_frame_hook = br_handle_frame;
|
|
|
|
br_fdb_get_hook = br_fdb_get;
|
|
br_fdb_put_hook = br_fdb_put;
|
|
|
|
register_netdevice_notifier(&br_device_notifier);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static void __exit br_deinit(void)
|
|
{
|
|
llc_sap_close(br_stp_sap);
|
|
|
|
#ifdef CONFIG_BRIDGE_NETFILTER
|
|
br_netfilter_fini();
|
|
#endif
|
|
unregister_netdevice_notifier(&br_device_notifier);
|
|
brioctl_set(NULL);
|
|
|
|
br_cleanup_bridges();
|
|
|
|
synchronize_net();
|
|
|
|
br_fdb_get_hook = NULL;
|
|
br_fdb_put_hook = NULL;
|
|
|
|
br_handle_frame_hook = NULL;
|
|
br_fdb_fini();
|
|
}
|
|
|
|
EXPORT_SYMBOL(br_should_route_hook);
|
|
|
|
module_init(br_init)
|
|
module_exit(br_deinit)
|
|
MODULE_LICENSE("GPL");
|
|
MODULE_VERSION(BR_VERSION);
|